Gtk Notebook
The Python
This is the code to add a tab to the notebook. The object self.notebook is created in the init method. When the menu File > New is activated the following code is ran.
def on_gtk_new_activate(self, menuitem, data=None):
# debugging message
print 'File New selected'
# create a label for the tab and using get_n_pages() to find out how
# many pages there is so the next page has a sequential number.
self.label1 = gtk.Label('Page ' + str(self.notebook.get_n_pages() + 1))
# create a label to put into the page
self.label2 = gtk.Label('Hello World')
# If you don't show the contents of the tab it won't show up
self.label2.show()
# append a page with label5 as the contents and label5 as the tab
self.notebook.append_page(self.label2, self.label1)
The code to tell what tab we are on is the following. The switch page signal is activated when a page is changed. It passes the notebook, the page, and the page number. This can be used to do different things based on what the current tab is.
def on_notebook1_switch_page(self, notebook, page, page_num, data=None):
self.tab = notebook.get_nth_page(page_num)
self.label = notebook.get_tab_label(self.tab).get_label()
self.message_id = self.statusbar.push(0, self.label)
The following is the complete python program.
#!/usr/bin/env python
import gtk
class Buglump:
def __init__(self):
self.gladefile = 'glade5.glade'
self.builder = gtk.Builder()
self.builder.add_from_file(self.gladefile)
self.builder.connect_signals(self)
self.window = self.builder.get_object('window1')
self.aboutdialog = self.builder.get_object('aboutdialog1')
self.statusbar = self.builder.get_object('statusbar1')
self.notebook = self.builder.get_object('notebook1')
# this updates the status bar with the current page
self.on_notebook1_switch_page(self.notebook,'',0)
self.window.show()
def on_window1_destroy(self, object, data=None):
print 'quit with cancel'
gtk.main_quit()
def on_gtk_quit_activate(self, menuitem, data=None):
print 'quit from menu'
gtk.main_quit()
def on_display_status_activate(self, menuitem, data=None):
print 'updated status bar'
self.message_id = self.statusbar.push(0, 'Updated Status Bar')
def on_clear_status_activate(self, menuitem, data=None):
print 'cleared status bar'
self.statusbar.remove_message(0, self.message_id)
def on_gtk_about_activate(self, menuitem, data=None):
print 'help about selected'
self.response = self.aboutdialog.run()
self.aboutdialog.hide()
def on_gtk_new_activate(self, menuitem, data=None):
print 'File New selected'
self.label1 = gtk.Label('Page ' + str(self.notebook.get_n_pages() + 1))
self.label2 = gtk.Label('Hello World')
self.label2.show()
self.notebook.append_page(self.label2, self.label1)
def on_notebook1_switch_page(self, notebook, page, page_num, data=None):
self.tab = notebook.get_nth_page(page_num)
self.label = notebook.get_tab_label(self.tab).get_label()
self.message_id = self.statusbar.push(0, self.label)
if __name__ == '__main__':
main = Buglump()
gtk.main()
The following images show what we get when we run the program.
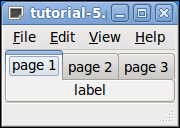
After clicking on File > New we see the new tab added.
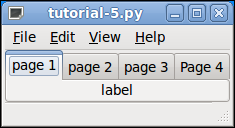
After clicking on the new tab we see the contents of our label.
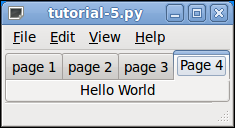
That’s all for this one and we couldn’t stand it any more and had to say Hello World… and we actually did something useful which is amazing.