Gtk ComboBox
The Python Side
Gtk ComboBox
The Gtk ComboBox is a bit complicated to use at first until you understand the related components needed.

Gtk ListStore
First we create an instance of the ListStore. The ListStore will have two columns with the first one being an integer and the second one a string. There are more complicated ways to do this but I like the KISS method.
self.liststore = gtk.ListStore(int,str)
Next we append our data to the ListStore. Each time we are adding two items the first is an integer for the row and the second is the text we want displayed on that row. The items we append to the ListStore in the form of a python list as indicated
self.liststore.append([0,"Select an Item:"])
self.liststore.append([1,"Row 1"])
self.liststore.append([2,"Row 2"])
self.liststore.append([3,"Row 3"])
self.liststore.append([4,"Row 4"])
self.liststore.append([5,"Row 5"])
Building the ComboBox
First we get an instance of our ComboBox which has the name combobox1 from the builder.
self.combobox = self.builder.get_object("combobox1")
Next we set the ListStore as the Model of the ComboBox
self.combobox.set_model(self.liststore)
Next we create an instance of the Gtk CellRendererText object. And using that and a little magic I think we pack the Gtk CellRendererText into the combobox. The only thing I know about line three is the number indicates what column is displayed and the first column is 0.
self.cell = gtk.CellRendererText()
self.combobox.pack_start(self.cell, True)
self.combobox.add_attribute(self.cell, 'text', 1)
And last we set the active row for the combobox. When this happens it also triggers the on_combobox1_changed event.
self.combobox.set_active(0)
ComboBox Changed
The following code is executed when ever the combobox is changed. When the Handler is called it is passed the name of the widget. We use this to be able to change things.
def on_combobox1_changed(self, widget, data=None):
# get the index of the changed row
self.index = widget.get_active()
# get the model
self.model = widget.get_model()
# retrieve the item from column 1
self.item = self.model[self.index][1]
# debuggin print statements
print "ComboBox Active Text is", self.item
print "ComboBox Active Index is", self.index
# set the text of the label to match the selected item
self.builder.get_object("label1").set_text(self.item)
The Python Code
#!/usr/bin/env python
try:
import pygtk
pygtk.require('2.0')
except:
pass
try:
import gtk
import gtk.glade
except:
print('GTK not available')
sys.exit(1)
class Buglump:
def __init__(self):
self.builder = gtk.Builder()
self.builder.add_from_file("glade6.glade")
self.builder.connect_signals(self)
# the liststore
self.liststore = gtk.ListStore(int,str)
self.liststore.append([0,"Select an Item:"])
self.liststore.append([1,"Row 1"])
self.liststore.append([2,"Row 2"])
self.liststore.append([3,"Row 3"])
self.liststore.append([4,"Row 4"])
self.liststore.append([5,"Row 5"])
# the combobox
self.combobox = self.builder.get_object("combobox1")
self.combobox.set_model(self.liststore)
self.cell = gtk.CellRendererText()
self.combobox.pack_start(self.cell, True)
self.combobox.add_attribute(self.cell, 'text', 1)
self.combobox.set_active(0)
self.window = self.builder.get_object("window1")
self.window.show()
def on_combobox1_changed(self, widget, data=None):
self.index = widget.get_active()
self.model = widget.get_model()
self.item = self.model[self.index][1]
print "ComboBox Active Text is", self.item
print "ComboBox Active Index is", self.index
self.builder.get_object("label1").set_text(self.item)
def on_window1_destroy(self, object, data=None):
print "quit with cancel"
gtk.main_quit()
if __name__ == "__main__":
main = Buglump()
gtk.main()
Run the App
Open a terminal and change directory to the directory where your files are. Type in ls to see a list of the files. Type in chmod +x glade6.py to make the glade6.py file executable. Now to run the app type in ./glade6.py.
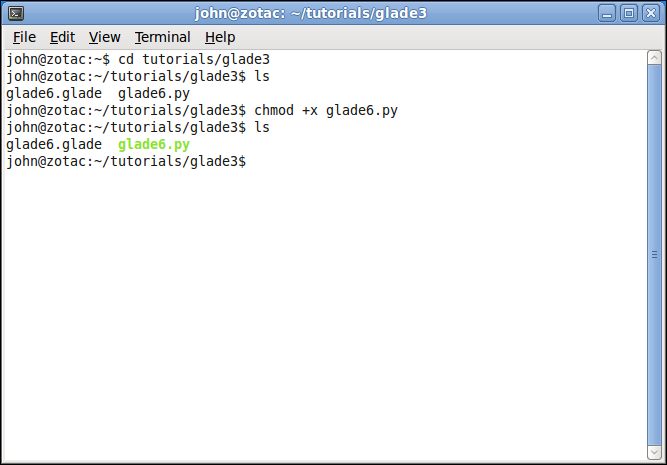
When you first start the application the on_combobox1_changed is ran because of the line self.combobox.set_active(0)
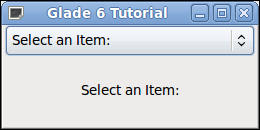
After selecting an item from the ComboBox the item text is mirrored in the label.

Next up is using a sqlite database to populate the ComboBox.