Inputs¶
An example using the P1-16ND3 input module with the P1AM-100 PLC to execute commands only once when an input is toggled.
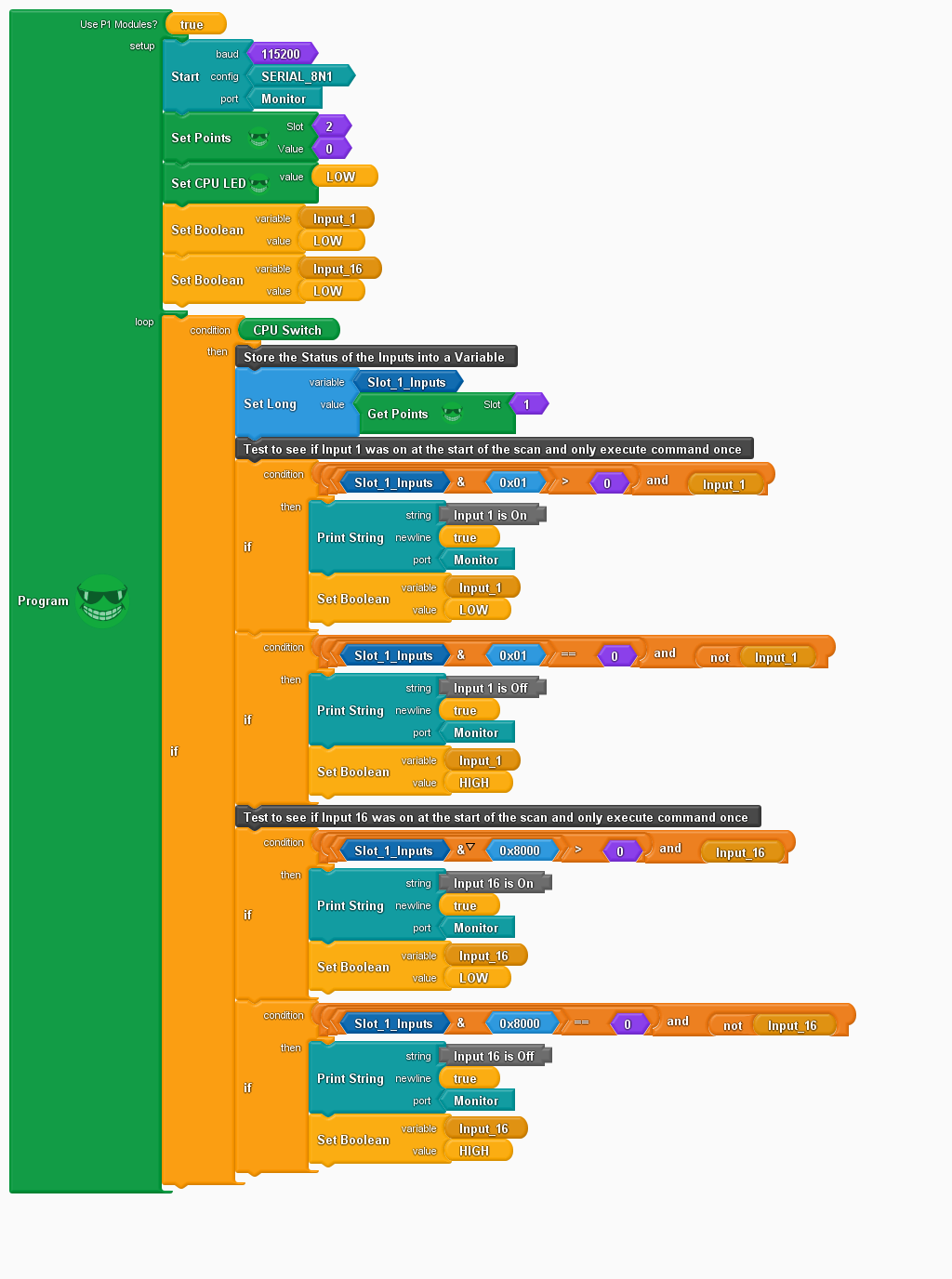
Starting from the top Use P1 Modules? is set to true so the backplane is powered.
Setup¶
Start serial communications.
If you have an output card(s) set the points to 0 (off).
Set the CPU LED Low (off)
Set two boolean variable Low (False). This is used to execute the commands only once and is set to Low so it matches the Off condition of the inputs.
Loop¶
The first condition is an if then test that uses the CPU Switch to start.
The first thing you want to do is to store the status of the inputs into a long variable. This is done so you only have to poll the input card once per scan to have the fastest program time.
There are two pairs of if then tests, one for input 1 and one for input 16.
The first if/then block tests to see if the input is on and the boolean variable is high (true). If that condition is true then print to the serial monitor and set the boolean variable to low. The next scan if the input is still on the condition is now false because the boolean is low.
The condition uses bit masking to look only at the first bit of the variable Slot_1_Inputs.
Left to right the condition is: ((Slot_1_Inputs variable and 0x01) is greater than 0) and Input_1 is high.
The second if/then block tests to see if the input is off and the boolean variable is low (false). If the condition is true then print to the serial monitor and set the boolean variable high. The next scan if the input is still off the condition is now false because the boolean is high.
Left to right the condition is: ((Slot_1_Inputs variable and 0x01) is equal to 0) and Input_1 is not high.
Bitwise And¶
Bitwise & (and) means if both bits are the same pass them through to the result. In this example we want to examine the first bit only so the bit mask is 0x01. If we wanted to check the third bit the mask would be 0x4 which doesn’t make sense if you’re not familiar with hexadecimal numbers.
Slot 1 Input 1 is Off
Binary
Slot_1_Inputs 1 0 0 1 1 0 1 0 1 0 1 1 1 0 0 0
And Hex 0x01 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1
Result 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
Slot 1 Input 1 is On
Binary
Slot_1_Inputs 1 0 0 1 1 0 1 0 1 0 1 1 1 0 0 1
And Hex 0x01 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1
Result 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1
16 Bit Numbers¶
Addressing a 16 bit number using hexadecimal bit masking you need to use the hex number with the bit mask.
Bit |
Binary |
Decimal |
Hex |
---|---|---|---|
1 |
0000000000000001 |
1 |
1 |
2 |
0000000000000010 |
2 |
2 |
3 |
0000000000000100 |
4 |
4 |
4 |
0000000000001000 |
8 |
8 |
5 |
0000000000010000 |
16 |
10 |
6 |
0000000000100000 |
32 |
20 |
7 |
0000000001000000 |
64 |
40 |
8 |
0000000010000000 |
128 |
80 |
9 |
0000000100000000 |
256 |
100 |
10 |
0000001000000000 |
512 |
200 |
11 |
0000010000000000 |
1024 |
400 |
12 |
0000100000000000 |
2048 |
800 |
13 |
0001000000000000 |
4096 |
1000 |
14 |
0010000000000000 |
8192 |
2000 |
15 |
0100000000000000 |
16384 |
4000 |
16 |
1000000000000000 |
32768 |
8000 |