Part 6e Adding Postgui HAL File
Glade Changes
Open up gui6.glade and change the Name: of hal_hbox1 to manual_box. Save the file and copy to the installed directory.
sudo cp gui6.glade /usr/share/linuxcnc
Postgui HAL File
Add a file to our configuration directory named postgui.hal and add the following to it.
# turn on and off manual tab of GUI net manual-enabled gui6.manual_box <= motion.motion-enabled
INI File Changes
-
Open up gui6.ini and add the following line to the '[HAL] section.
POSTGUI_HALFILE = postgui.hal
-
Add the following line to the [TRAJ] section.
NO_FORCE_HOMING = 1
Python Changes
-
Change the class init to take a second parameter.
change def __init__(self): to def __init__(self, inifile):
-
Add a method to our gui6 class to return the postgui hal file name
def postgui(self): inifile = linuxcnc.ini(self.ini_file) postgui_halfile = inifile.find("HAL", "POSTGUI_HALFILE") return postgui_halfile,sys.argv[2]
-
Change the run part of the python to check for a postgui file and execute it after the GUI loads.
change # run the program if __name__ == "__main__": app = gui6() gtk.main() to # run the program if __name__ == "__main__": if len(sys.argv) > 2 and sys.argv[1] == '-ini': print "2", sys.argv[2] app = gui6(sys.argv[2]) else: app = gui6() # load a postgui file if one is present in the INI file postgui_halfile,inifile = gui6.postgui(app) print "GUI6 postgui filename:",postgui_halfile if postgui_halfile: res = os.spawnvp(os.P_WAIT, "halcmd", ["halcmd", "-i",inifile,"-f", postgui_halfile]) if res: raise SystemExit, res gtk.main()
-
Copy the python file to the installed location.
sudo cp gui6 /usr/bin
Python Details
If you want to add a postgui hal file you must tell halcmd where the ini file is so it can parse the name of the postgui file.
Some of the weirdness of this code is because it is outside the gui6 class so you must call methods with the class name rather then using the usual self.
if __name__ == "__main__": if len(sys.argv) > 2 and sys.argv[1] == '-ini': print "ini", sys.argv[2] app = gui6(sys.argv[2]) else: app = gui6()
The sys.argv is what is passed to the program when it is launched. The LinuxCNC script adds the -ini to the string when it starts the main program. This is why when you run your GUI with linuxcnc path/to/ini/my.ini there is more than two sys.argv in the list. You can see what each one is by changing the position number in the print statement then running the GUI.
This looks for the a -ini switch that specifies the INI path. If no INI is passed then sys.argv will not be greater than 2 so the else does not specify an INI path.
Then it initializes an instance of the gui6 class called app, either with the specified INI path or without. Before we initialized an instance of the gui6 class without checking anything.
# load a postgui file if one is present in the INI file postgui_halfile,inifile = gui6.postgui(app) print "GUI 6 postgui filename:",postgui_halfile
This calls a method INSIDE the gui6 class so instead of self we use app (the instance name we gave it a couple lines ago) the method postgui() looks for the postgui filename in the INI file from the config that was started from and returns the postgui file name and the INI path
if postgui_halfile: res = os.spawnvp(os.P_WAIT, "halcmd", ["halcmd", "-i",inifile,"-f", postgui_halfile]) if res: raise SystemExit, res
So here we check to see if there was a postgui file name and if so we spawn another halcmd to load it. This time we allow INI substitution. again if there is an error we exit the program with an error message.
gtk.main()
Of course gtk.main() starts monitoring signals from our program.
Test Run
When you run the GUI from the desktop launcher you should be able to cycle the E-Stop and the Power buttons and see the Manual tab enabled. Notice the Pause button is disabled like you expect when not running a program. Even though you can load a program and the Run button works you can not run a program until the stepgens are added. Try to run a program and see for yourself what happens. Hint the error status is printed to the terminal.
Your GUI should look like this now.
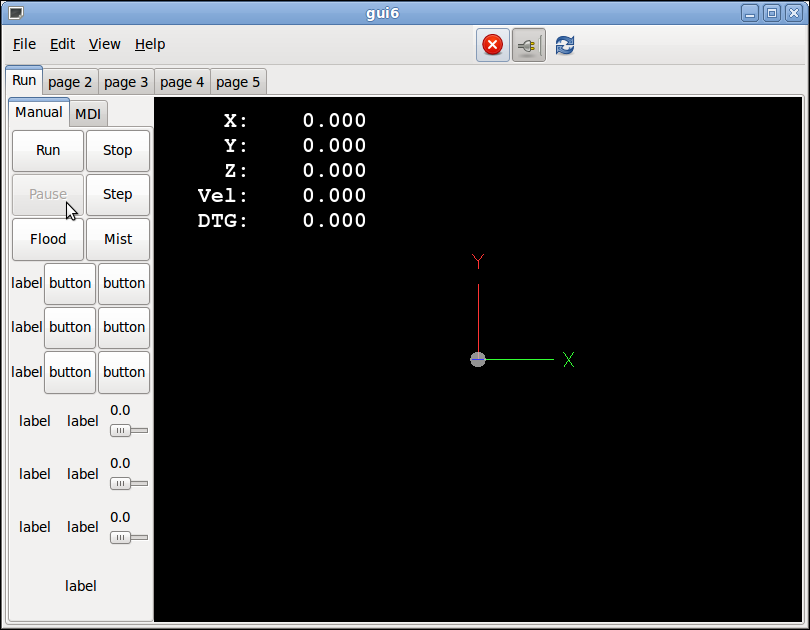