Part 6h Creating Jog Commands
Python Changes
-
Open up the gui6 python file and add the following to the gui6 class ini method.
def __init__(self, inifile): self.cnc = linuxcnc self.s = self.cnc.stat() self.c = self.cnc.command() self.ec = self.cnc.error_channel()
-
Starting with the X plus jog button change the methods to add a couple of things. When 'jog_x_plus is pressed we first see if the jog slider is greater than zero. If it is then we use command to jog the axis at the velocity shown on the slider. When the jog_x_plus is released we issue a command to stop the jogging action. We need to divide the IPM value by 60 to IPS which is what command expects.
# jogging def on_jog_x_plus_pressed(self, widget, data=None): print 'jog x plus pressed' if self.builder.get_object("jog_speed").get_value() > 0: self.c.jog(self.cnc.JOG_CONTINUOUS, 0, self.builder.get_object("jog_speed").get_value() / 60) def on_jog_x_plus_released(self, widget, data=None): print 'jog x plus released' self.c.jog(self.cnc.JOG_STOP, 0)
-
Save and copy the gui6 file to the installed location.
sudo cp gui6 /usr/bin
At this point you should be able to jog the X axis in the positive direction.
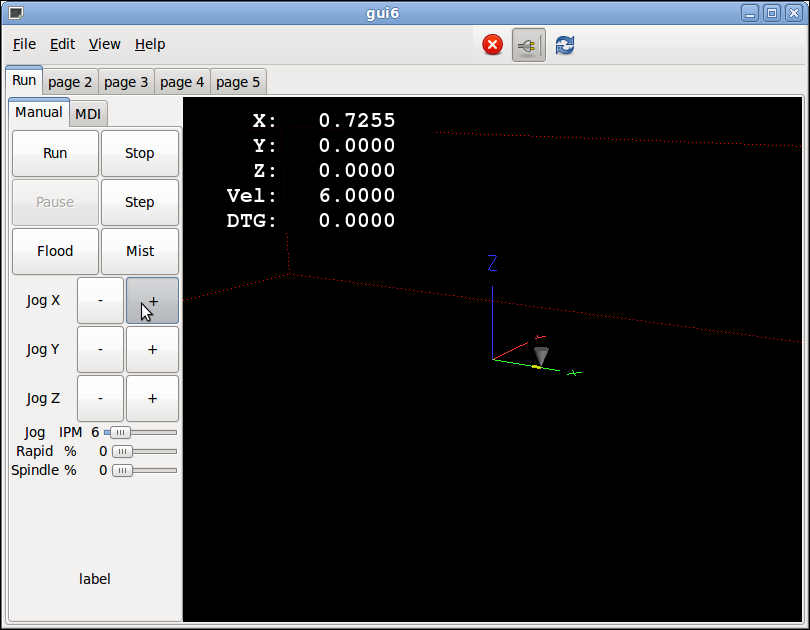
-
Lets add the rest of the jog commands to our methods. Notice that JOG_STOP is axis specific and if you don’t call JOG_STOP when you release the button that axis will continue to jog. The following is all the axis jog methods.
# jogging def on_jog_x_plus_pressed(self, widget, data=None): print 'jog x plus pressed' if self.builder.get_object("jog_speed").get_value() > 0: self.c.jog(self.cnc.JOG_CONTINUOUS, 0, self.builder.get_object("jog_speed").get_value() / 60) def on_jog_x_plus_released(self, widget, data=None): print 'jog x plus released' self.c.jog(self.cnc.JOG_STOP, 0) def on_jog_x_minus_pressed(self, widget, data=None): print 'jog x minus pressed' if self.builder.get_object("jog_speed").get_value() > 0: self.c.jog(self.cnc.JOG_CONTINUOUS, 0, -self.builder.get_object("jog_speed").get_value() / 60) def on_jog_x_minus_released(self, widget, data=None): print 'jog x minus released' self.c.jog(self.cnc.JOG_STOP, 0) def on_jog_y_plus_pressed(self, widget, data=None): print 'jog y plus pressed' if self.builder.get_object("jog_speed").get_value() > 0: self.c.jog(self.cnc.JOG_CONTINUOUS, 1, self.builder.get_object("jog_speed").get_value() / 60) def on_jog_y_plus_released(self, widget, data=None): print 'jog y plus released' self.c.jog(self.cnc.JOG_STOP, 1) def on_jog_y_minus_pressed(self, widget, data=None): print 'jog y minus pressed' if self.builder.get_object("jog_speed").get_value() > 0: self.c.jog(self.cnc.JOG_CONTINUOUS, 1, -self.builder.get_object("jog_speed").get_value() / 60) def on_jog_y_minus_released(self, widget, data=None): print 'jog y minus released' self.c.jog(self.cnc.JOG_STOP, 1) def on_jog_z_plus_pressed(self, widget, data=None): print 'jog z plus pressed' if self.builder.get_object("jog_speed").get_value() > 0: self.c.jog(self.cnc.JOG_CONTINUOUS, 2, self.builder.get_object("jog_speed").get_value() / 60) def on_jog_z_plus_released(self, widget, data=None): print 'jog z plus released' self.c.jog(self.cnc.JOG_STOP, 2) def on_jog_z_minus_pressed(self, widget, data=None): print 'jog z minus pressed' if self.builder.get_object("jog_speed").get_value() > 0: self.c.jog(self.cnc.JOG_CONTINUOUS, 2, -self.builder.get_object("jog_speed").get_value() / 60) def on_jog_z_minus_released(self, widget, data=None): print 'jog z minus released' self.c.jog(self.cnc.JOG_STOP, 2)
-
Save and copy the gui6 file to the installed location.
sudo cp gui6 /usr/bin
At this point you should be able to jog all the axes.
